License
Each licence is custom post and data is stored inside metabox. Its strucured into two sections.
- Licence Settings
- Used Sites
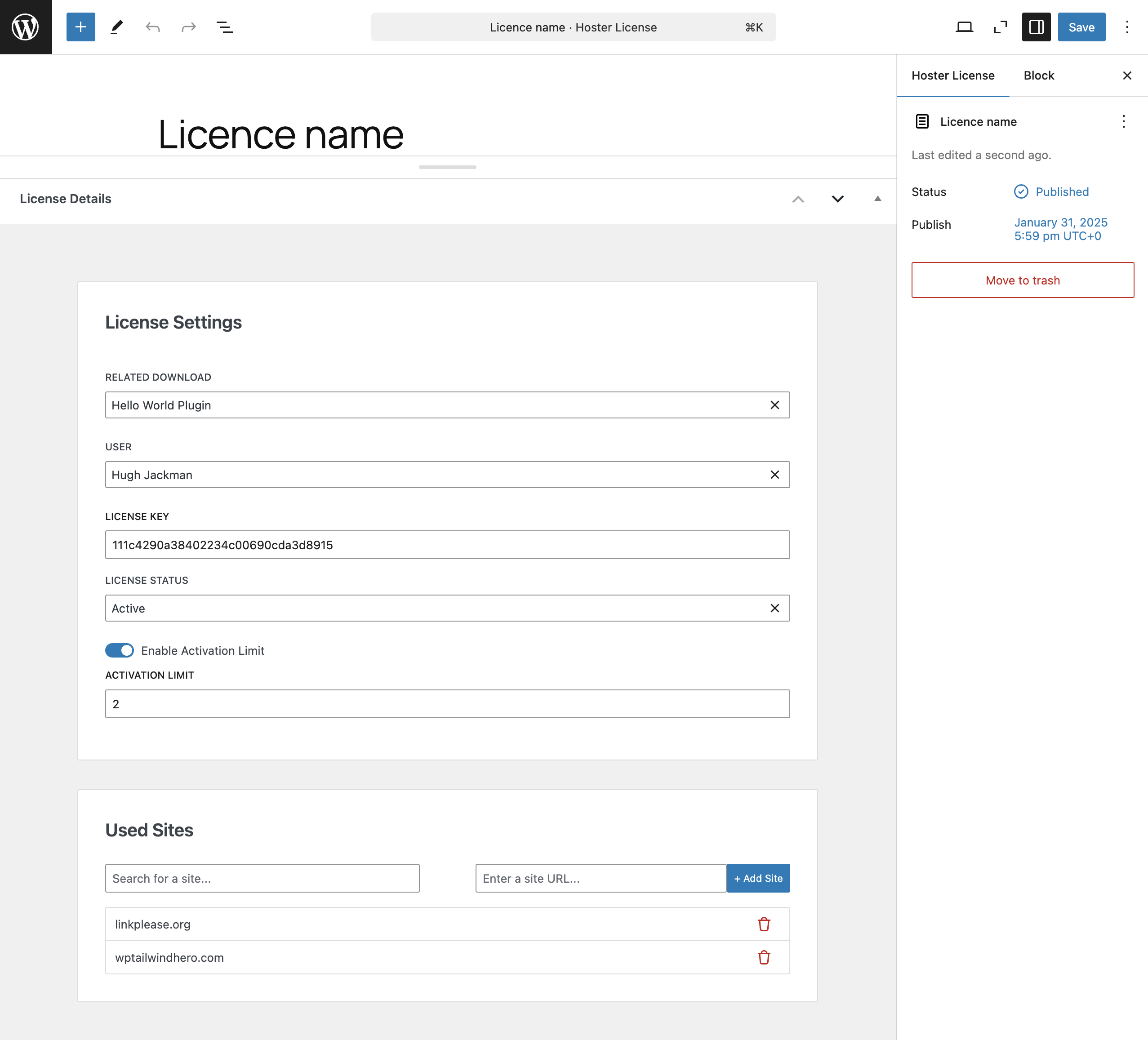
Note: This is not the payment flow. This is a hook to generate the license. With this approach, you can trigger it with WooCommerce, EDD, Stripe, or any other payment system.
Licence Settings
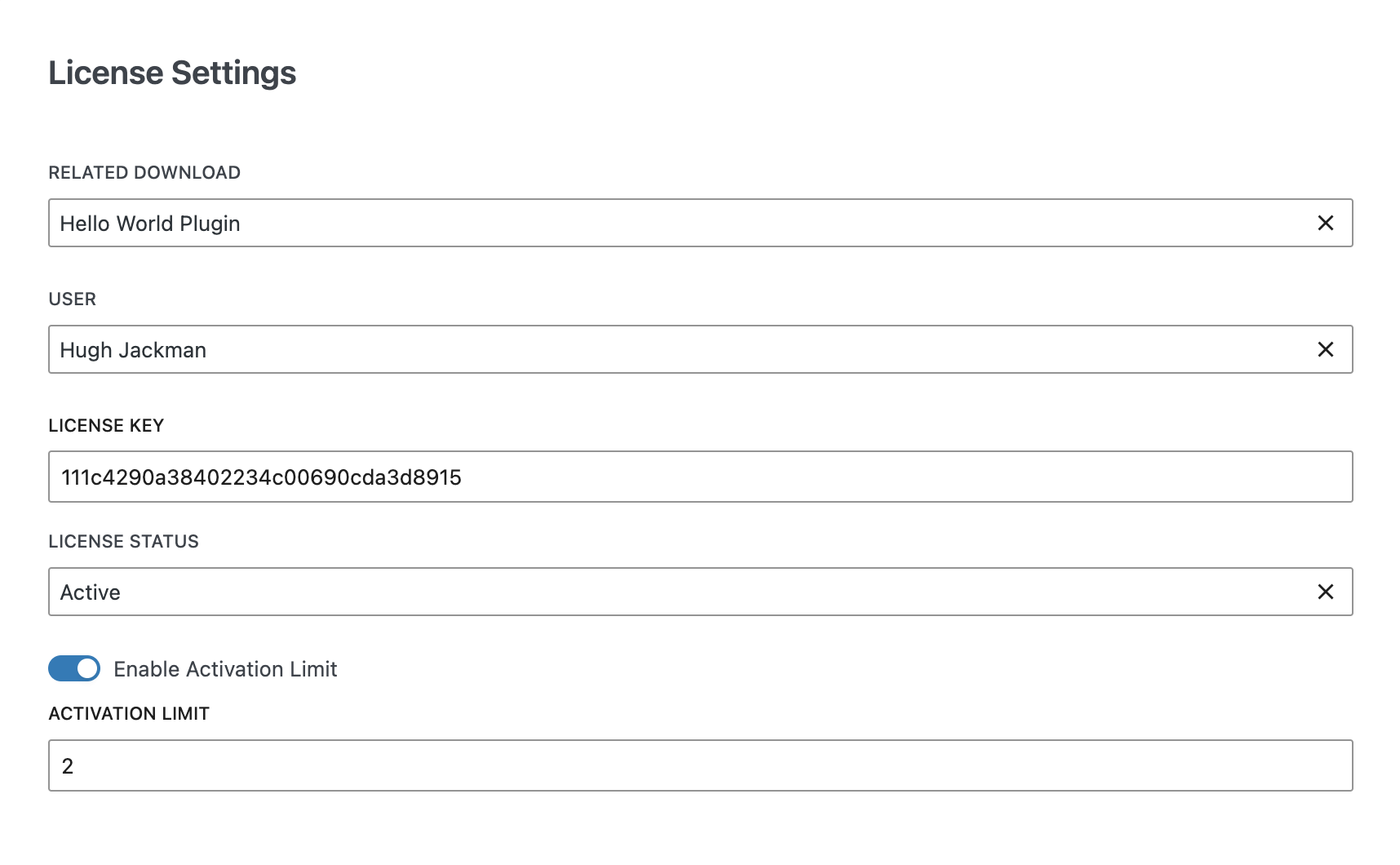
Related Download
The assigned plugin or theme using this license. You can change it manually if needed.
User
The assigned user for the plugin or theme using this license. You can change it manually if needed.
License Key
This is an autogenerated key, and you cannot change it.
License Status
We have three statuses here:
- Active, Everything is okay, and the user is valid to use it.
- Expired, If you are using recurring payments, the license can become expired.
- Invalid, Sometimes, payments fail, but the license may still be triggered.
We have enabled the option to switch it manually as well.
Enable Activation Limit
By default, it’s set to unlimited. You can enable it to get an extra field to enter a number to set a limit.
Activation Limit
Set the number of websites on which the license can be activated.
Expiry Date
Set the expiry date for the license, we have written a CRON job which will set the license status to “expired” automatically if the expiry date is set.
We have also added a WP-CLI command if you want to manually trigger the CRON “wp hoster_expire_licenses”
Used Sites
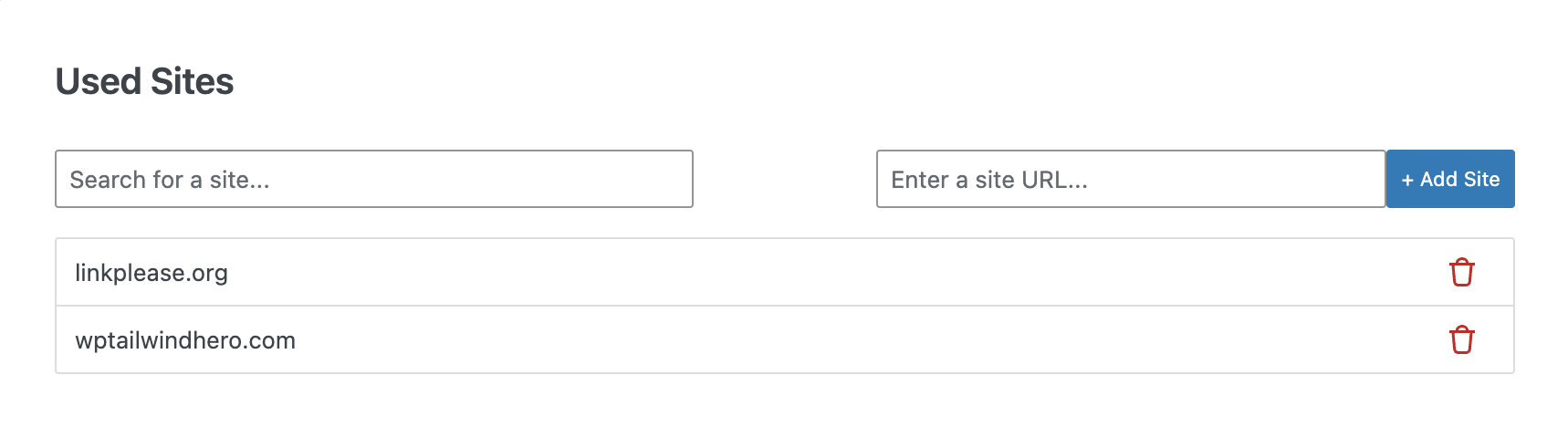
This is an overview of all the websites where the current license is activated. You can also manually add or delete websites from the list.
Licence Implementation
To implement the license handler inside your plugin or theme, navigate to the host resources and choose your product so we can generate the exact code for you.
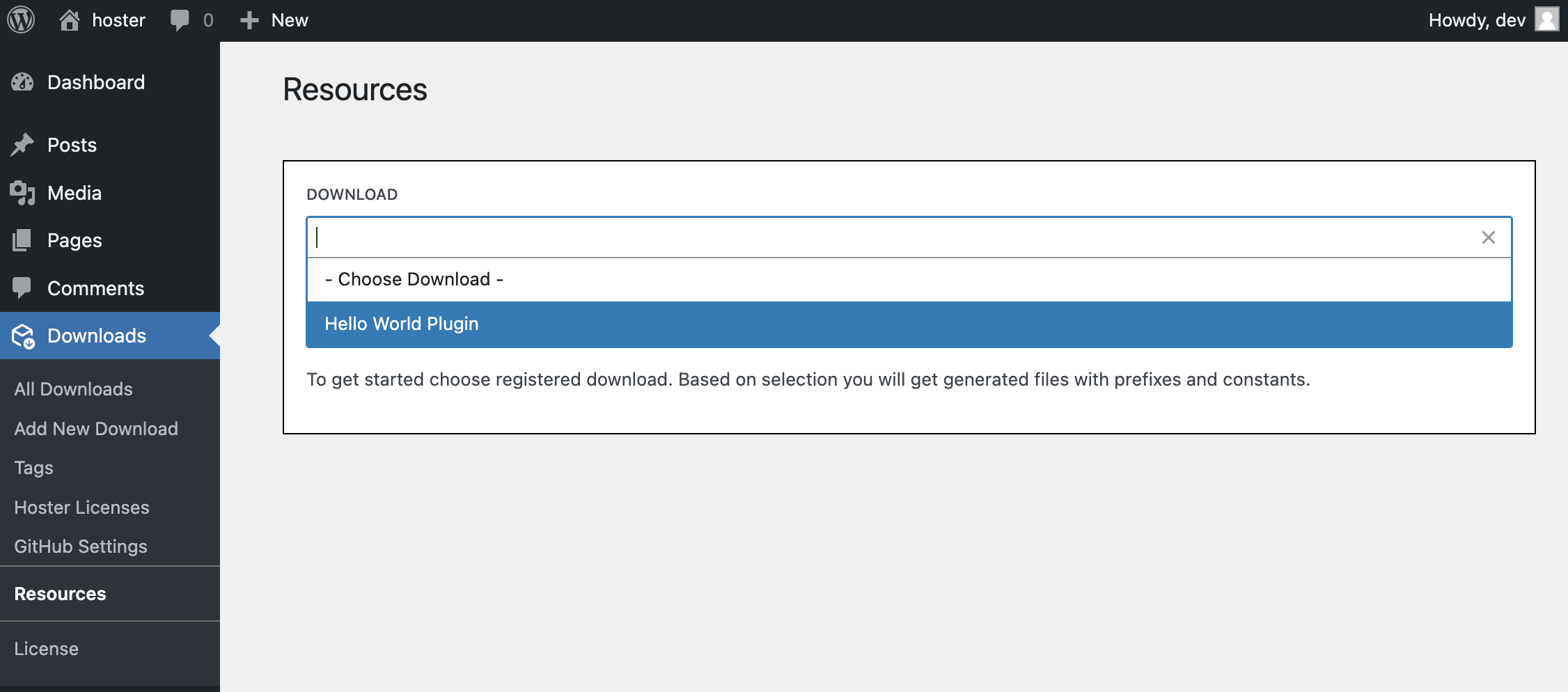
Once the plugin is selected, navigate to the second tab, “Updater,” and the ready-made code will be generated. Use the copy function to copy the code and paste it under the ./inc/updater.php file.
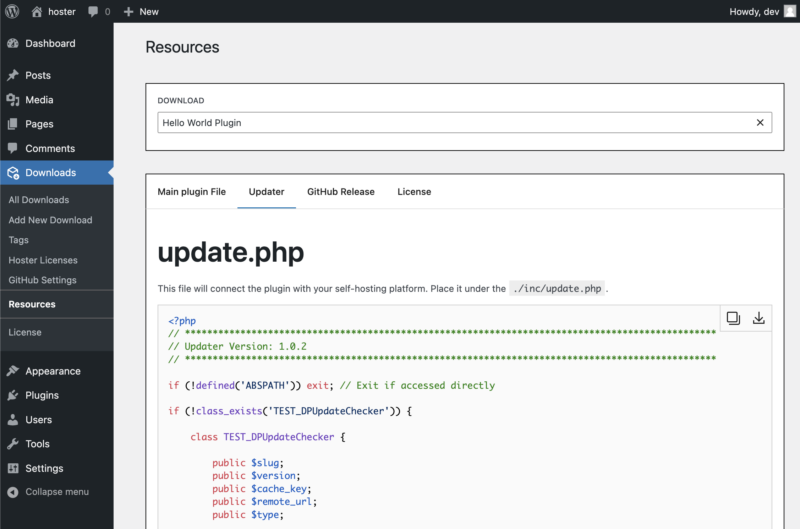
Create License Using Hook
Following is the function that you can use to create license using hook when using Woocommerce or any tool to get the payments.
/*
* Example usage of the action hook
* $response = apply_filters('hoster_create_new_license', null, 27, 1, 'active', true, 5, '2025-02-13');
*/
/*
* Creates a new license and returns the license key & ID.
*
* @param null - this should always be null
* @param int $download_id - The related download post ID.
* @param int $user_id - The WordPress user ID.
* @param string $status - The license status (active/expired).
* @param bool $enable_activation_limit - Whether activation limit is enabled (true/false).
* @param int $activation_limit - The max activations allowed.
* @param string $expiry_date - Expiry date if you want to set it for auto expire of license.
*
* @return array - Success or error response.
*/
Activate or Deactivate License
Following is the function that you can use to activate or deactivate license on your hosted plugin.
/*
* @param download id
* @param license key
* @param site URL
*/
$plugin_prefix = 'HELLOPLUGIN';
require_once (constant($plugin_prefix . '_PATH') . 'inc/hoster-license-handler.php');
$className = $plugin_prefix . '_LicenseHandler';
$license_handler = new $className();
// Activate License Example
/*
* Activates the licnese and send response array
*
* @param success - boolean true or false
* @param message - string
* @param license_id - int
* @param expiry_date - string
* @param token - string, this will be used to pass to get the info.json file
* @param used_activations - int
* @param remaining activations - int
*
* @return array - Success or error response.
*/
$response = $license_handler->activate_license(27, 'YOUR-LICENSE-HERE', get_site_url());
echo "<pre>"; print_r($response); echo "</pre>";
// Deactivate License Example
/*
* Deactivates the licnese and send response array
*
* @param success - boolean true or false
* @param message - string
* @param license_id - int
*
* @return array - Success or error response.
*/
$response = $license_handler->deactivate_license(27, 'YOUR-LICENSE-HERE', get_site_url());
echo "<pre>"; print_r($response); echo "</pre>";
// Check License Example
/*
* Checks the licnese and send response array
*
* @param success - boolean true or false
* @param message - string
* @param license_id - int
* @param status - string active/invalid/expired
* @param expiry_date - string
* @param site_activated - string
* @param used_activations - int
* @param remaining activations - int
*
* @return array - Success or error response.
*/
$response = $license_handler->check_license(27, 'YOUR-LICENSE-HERE', get_site_url());
echo "<pre>"; print_r($response); echo "</pre>";